主题
Skip to content
说明
为了方便后续对产品进行优化,需要添加操作数据埋点的逻辑
统计接口的核心代码在 ReportManager
中
大部分基础事件,如启动,时长,广告播放,崩溃等事件,SDK 内部都已做处理,部分和游戏玩法强关联的事件,需要 CP 在集成过程中自主上报。
WARNING
如果 CP 没有使用组件式视频激励广告,在集成激励视频广告时,请参考 VideoButton 示例 中的内容,将视频相关的上报,集成到自定义的视频按钮行为中
必须上报的事件有两部分,一部分是无玩法参数接口,只用作视频按钮点击率统计;另一部分是有玩法参数接口,是用于统计游戏事件和单个视频点的情况。
无玩法参数接口
ReportManager.I.TrackVideo(1);
视频按钮时(灰色不可点击)创建上报
ReportManager.I.TrackVideo(2);
广告请求成功(按钮可点击)时上报
ReportManager.I.TrackVideo(3);
用户点击按钮时上报
有玩法参数接口
ReportManager.I.NewReportVideoClick
用户点击视频按钮时
ReportManager.I.NewReportVideoUnable
视频按钮不可点击
ReportManager.I.NewReportVideoShow
视频按钮展示时,视频有填充
ReportManager.I.NewReportVideoNoAds
视频按钮展示时,视频无填充
ReportManager.I.NewReportVideoPlay
视频播放时
ReportManager.I.NewReportVideoSuccess
视频播放成功
ReportManager.I.NewReportVideoFail
视频播放失败
可以参考示例中的内容,来集成统计模块
接口
示例
C#
using System.Collections;
using System.Collections.Generic;
using ZMYSDK;
using ZMYSDK.Report;
using ZMYSDK.Report.JsonProperties;
using UnityEngine;
using UnityEngine.UI;
public class reportTest : MonoBehaviour
{
void Start()
{
//启动游戏应该先上报玩法开始
ReportManager.I.StartMineGame();
}
//再正式进入游戏核心玩法前,应该选择不同的玩法。也就是不同的模式,同一时间只存在一种模式
#region 模式上报
/// <summary>
/// 开始关卡模式
/// </summary>
public void mode_level(){ReportManager.I.StartGameModel("Level"); }
public void mode_PVP() { ReportManager.I.StartGameModel("PVP"); }//开始一个模式,会自动停掉上一个模式
public void mode_Customize() { ReportManager.I.StartGameModel("Customize"); }
public void mode_quit() { ReportManager.I.StartGameModel("None"); }//特殊标记为None,表示退出当前模式
#endregion
/*有关卡概念的游戏,应该进行关卡相关上报。
关卡上报分为:开始----过程中----结束。过程中的上报必须在关卡开始和结束期间进行上报。
否则会触发上报不合规,无法正常上报
关卡复活上报只能在关卡失败上报后进行上报
关卡退出上报不是关卡结束上报,关卡退出表示关卡流程被挂起,用户会再次进入挂起的关卡,并触发关卡继续上报。
*/
#region 关卡上报
/// <summary>
/// 关卡开始上报--单个关卡上报前 最开始的上报
/// </summary>
/// <param name="input"></param>
public void lv_GameStart(InputField input)
{
game_start data= ReportManager.I.GetNewReportJsonData<game_start>();
int lv = 1;
int.TryParse(input.text, out lv);
data.mode_level = lv;
ReportManager.I.NewReportGameStart(data);
}
//关卡退出上报-关卡过程中的上报
public void lv_GameQuit()
{
ReportManager.I.NewReportGameQuit();
}
//关卡进度上报-关卡过程中的上报
public void lv_GameProgress()
{
game_progress data = ReportManager.I.GetNewReportJsonData<game_progress>();
data._current_gold++;
ReportManager.I.NewReportGameProgress(data);
}
//关卡犯规上报-关卡过程中的上报
public void lv_GameFoul()
{
game_foul data = ReportManager.I.GetNewReportJsonData<game_foul>();
data.activity_name = "游戏犯规1";
ReportManager.I.NewReportGameFoul(data);
}
//关卡失败上报-关卡结束上报
public void lv_GameFail()
{
game_fail data = ReportManager.I.GetNewReportJsonData<game_fail>();
ReportManager.I.NewReportGameFail(data);
}
//关卡完成上报-关卡结束上报
public void lv_GameComplete()
{
game_complete data = ReportManager.I.GetNewReportJsonData<game_complete>();
ReportManager.I.NewReportGameComplete(data);
}
//关卡平局上报-关卡结束上报
public void lv_GameDraw()
{
game_draw data = ReportManager.I.GetNewReportJsonData<game_draw>();
ReportManager.I.NewReportGameDraw(data);
}
//关卡复活上报-一种特殊的上报
public void lv_GameRevive()
{
game_revive data = ReportManager.I.GetNewReportJsonData<game_revive>();
ReportManager.I.NewReportGameRevive(data);
}
#endregion
}
VideoButton 示例
C#
using System.Collections;
using System.Collections.Generic;
using ZMYSDK;
using ZMYSDK.Report;
using ZMYSDK.Util.Event;
using ZMYSDK.Util.Lang;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.Events;
public class VideoButton : MonoBehaviour
{
[SerializeField]
private BtnShowType m_btnType = BtnShowType.SingleShow;
[SerializeField]
private VideFlag m_flag;
public UnityEvent<bool> m_videoPlayCallBack;
public UnityEvent<bool> m_btnOnClick;
/// <summary>按钮的三种状态</summary>
private BtnState btnstate { get; set; }
BtnReportState btnReportState = BtnReportState.None;
/*===========上报相关的三个参数=============*/
[SerializeField]
private string m_Video_name;
[SerializeField]
private string m_Video_param1;
[SerializeField]
private string m_Video_param2;
#region 对外属性
public BtnShowType BtnType
{
get { return m_btnType; }
set { m_btnType = value; }
}
public VideFlag Flag
{
get { return m_flag; }
set { m_flag = value; }
}
public string Video_name
{
get { return m_Video_name; }
set { m_Video_name = value; }
}
public string Video_param1
{
get { return m_Video_param1; }
set { m_Video_param1 = value; }
}
public string Video_param2
{
get { return m_Video_param2; }
set { m_Video_param2 = value; }
}
#endregion
/// 自动上报视频加载时上报 </summary>
public bool AutoCallBtnShow = true;
void Awake()
{
#if UNITY_EDITOR
if (!UnityEditor.EditorApplication.isPlaying)
return;
#endif
if (m_btnType == BtnShowType.Condition)
{
gameObject.SetActive(false);
}
}
void Start()
{
#if UNITY_EDITOR
if (!UnityEditor.EditorApplication.isPlaying)
return;
#endif
MsgDispatcher.AddEventListener(GlobalEventType.VideoButtonStatus, VideoButtonStatus);
//条件视频按钮不走这条逻辑
if (m_btnType == BtnShowType.Condition)
return;
bool IsReady = ZMYSDKManager.I.IsVideoReady();
SetButtonState(IsReady ? BtnState.Active : BtnState.ActiveNotClick);
}
/// <summary>
/// 由于打开UI默认隐藏,导致OnEnable会调用2次。所以对OnEnable进行0.5s的间隔判断
/// </summary>
private float OnEnableWeekTime;
void OnEnable()
{
if (Time.time < OnEnableWeekTime)
{
Debug.Log("激励视频多次激活,CD未好");
return;
}
OnEnableWeekTime = Time.time + 0.5f;
#if UNITY_EDITOR
if (!UnityEditor.EditorApplication.isPlaying)
return;
#endif
//视频上报
if (AutoCallBtnShow)
VideoBtnShow(true);
if (m_btnType == BtnShowType.Condition)
return;
//过1S后上报创建
StartCoroutine(DelayReport(1));
}
private bool isDestroy = false;
protected void OnDestroy()
{
isDestroy = true;
m_videoPlayCallBack = null;
m_btnOnClick = null;
MsgDispatcher.RemoveEventListener(GlobalEventType.VideoButtonStatus, VideoButtonStatus);
}
private void Update()
{
CheckClickVideoBtnCD();
}
#region 基础的按钮点击事件
const int PLAYVIDEOCD = 5;
const int CLICKBTNCD = 1;
static bool m_isPlayVideo = false;
static float m_PlayVideoTimer;
bool m_isClickVideo = false;
float m_ClickVideoTimer;
void CheckClickVideoBtnCD()
{
if (m_isClickVideo)
{
//开始清空点击倒计时
if (Time.unscaledTime > m_ClickVideoTimer)
m_isClickVideo = false;
}
if (m_isPlayVideo)
{
//开始清视频播放倒计时
if (Time.unscaledTime > m_PlayVideoTimer)
m_isPlayVideo = false;
}
}
/// <summary>
/// 点击视频按钮
/// </summary>
public void OnClcikVideoBtn()
{
#if UNITY_EDITOR
if (!UnityEditor.EditorApplication.isPlaying)
return;
#endif
if (m_isPlayVideo)//视频播放内置CD
{
Debug.Log($"目前正处于视频播放CD中:{m_PlayVideoTimer - Time.unscaledTime}");
return;
}
if (m_isClickVideo)//视频点击内置CD
{
Debug.Log($"目前正处于视频点击CD中{m_ClickVideoTimer - Time.unscaledTime}");
return;
}
//CLog.Log("点击了视频按钮");
m_isClickVideo = true;
m_ClickVideoTimer = Time.unscaledTime + CLICKBTNCD;
VideoClickReport();
m_btnOnClick?.Invoke(btnstate == BtnState.Active);
if (btnstate == BtnState.Active)
{
// CLog.Log("播放视频");
m_isPlayVideo = true;
m_PlayVideoTimer = Time.unscaledTime + PLAYVIDEOCD;
ReportManager.I.NewReportVideoPlay(m_Video_name, m_Video_param1, m_Video_param2);
ZMYSDKManager.I.ShowVide(m_flag, VideoCallBack);
}
else
{
//广告没准备好
ZMYSDKManager.I.Sdk.ShowToast(SdkLang.I.Get("sdk_noadstips"));
}
}
/// <summary>
/// 视频播放回调
/// </summary>
/// <param name="i"></param>
void VideoCallBack(bool i)
{
if (m_btnType == BtnShowType.MultipleShow)
{
btnReportState = BtnReportState.None;
if (!isDestroy && gameObject.activeSelf)
{
//过1S后重新统计上报
StartCoroutine(DelayReport(1));
}
}
if (i)
ReportManager.I.NewReportVideoSuccess(m_Video_name, m_Video_param1, m_Video_param2);
else//上报失败
ReportManager.I.NewReportVideoFail(m_Video_name, m_Video_param1, m_Video_param2);
m_videoPlayCallBack?.Invoke(i);
}
#endregion
#region 按钮状态变更
void VideoButtonStatus(object[] Args)
{
if (null == this.gameObject || isDestroy)
{
MsgDispatcher.RemoveEventListener(GlobalEventType.VideoButtonStatus, VideoButtonStatus);
return;
}
if (Args == null || Args.Length <= 0)
return;
int status = (int)Args[0];
switch (status)
{
case 0:
SetButtonState(BtnState.Hide);
break;
case 1:
SetButtonState(BtnState.ActiveNotClick);
break;
case 2:
SetButtonState(BtnState.Active);
break;
}
}
void SetButtonState(BtnState btnState)
{
this.btnstate = btnState;
switch (btnstate)
{
case BtnState.Hide:
gameObject.SetActive(false);
break;
case BtnState.ActiveNotClick:
break;
case BtnState.Active:
//视频准备好了,检查下是否需要上报请求成功
if (btnReportState == BtnReportState.Create)
VideoIsReadyReport();
break;
default:
break;
}
}
#endregion
#region 视频的上报
/// <summary>
/// 创建上报
/// </summary>
void CreateVideoReport()
{
ReportManager.I.TrackVideo(1); btnReportState = BtnReportState.Create;
}
/// <summary>
/// 请求成功上报
/// </summary>
void VideoIsReadyReport()
{
ReportManager.I.TrackVideo(2); btnReportState = BtnReportState.Ready;
}
/// <summary>
/// 视频点击上报
/// </summary>
void VideoClickReport()
{
ReportManager.I.TrackVideo(3);
ReportManager.I.NewReportVideoClick(m_Video_name, m_Video_param1, m_Video_param2);
}
IEnumerator DelayReport(int n)
{
yield return new WaitForSeconds(n);
CreateVideoReport();
//如果视频能够播放,那么就立刻上报请求成功
if (ZMYSDKManager.I.Sdk.IsVideoReadyStatic())
{
VideoIsReadyReport();
}
}
/// <summary>
/// 视频按钮显示到屏幕上了
/// </summary>
/// <param name="IsAvailable"></param>
public void VideoBtnShow(bool IsAvailable)
{
bool IsReady = ZMYSDKManager.I.Sdk.IsVideoReadyStatic();
if (IsAvailable)
{
if (IsReady)
{
ReportManager.I.NewReportVideoShow(m_Video_name, m_Video_param1, m_Video_param2);
}
else
{
ReportManager.I.NewReportVideoNoAds(m_Video_name);
}
}
else
{
ReportManager.I.NewReportVideoUnable(m_Video_name);
}
}
#endregion
}
点我快速对接
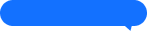

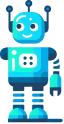