主题
Skip to content
说明
支付模块提供了完善的支付功能
在接入支付模块之前,请确保已经和商务对接,申请到了各个市场的支付商品 ID
部分市场在申请商品 ID 之前,需要出一个申请支付 ID 的市场包提审,审核通过后才能申请到 ID
可以参考示例中的内容,来集成支付模块
接口
示例
C#
using System.Collections;
using System.Collections.Generic;
using System.Runtime.Serialization;
using ZMYSDK;
using UnityEngine;
using UnityEngine.UI;
public class IapTest : MonoBehaviour
{
#if UNITY_ANDROID
/// 金币-消耗型商品
private const string globKey = "com.test.test.test.pigbank1";
/// vip--非消耗型商品
private const string vipKey = "com.test.test.test.noad";
/// 月卡--订阅型商品
private const string monthlyCardKey = "test.test1";
#else
/// 金币-消耗型商品
private const string globKey = "com.test.test.test.pigbank";
/// vip--非消耗型商品
private const string vipKey = "com.test.test.test.noad";
/// 月卡--订阅型商品
private const string monthlyCardKey = "test.tset";
#endif
private int m_glob;
private bool m_vip;
private int m_mcState;
private string m_mcExpiresDate;
public Text m_glotxt;
public Toggle m_vipToggle;
public Text m_monthlyCardtxt;
public GameObject m_storePlan;
public GameObject m_loading;
public Text m_resultTxt;
public GameObject m_azStore;
public GameObject m_iosStore;
void Awake()
{
#if UNITY_ANDROID
m_azStore.SetActive(true);
m_iosStore.SetActive(false);
#else
m_azStore.SetActive(false);
m_iosStore.SetActive(true);
#endif
//读取之前缓存的值
m_glob = PlayerPrefs.GetInt(globKey,0);
m_vip = PlayerPrefs.GetInt(vipKey, 0) == 1;
m_mcState = PlayerPrefs.GetInt(monthlyCardKey,2);
m_mcExpiresDate = PlayerPrefs.GetString(monthlyCardKey+"Time");
ReshUI();
}
void ReshUI()
{
m_glotxt.text = "金币数量:"+m_glob.ToString();
m_vipToggle.isOn = m_vip;
//订阅商品只处理1 和2 其他情况维持默认
if (m_mcState == 1)
{
m_monthlyCardtxt.text = $"月卡用户,时间:[{m_mcExpiresDate}]";
}
else if (m_mcState == 2)
{
m_monthlyCardtxt.text = "订阅已过期";
}
}
// Start is called before the first frame update
void Start()
{
//使用内购前,要先初始化
ZMYSDKManager.I.InitIap(new List<ProductData>()
{
new ProductData(){productID = globKey,productType = ProductType.Consumable},//金币
new ProductData(){productID = vipKey,productType = ProductType.NoConsumable},//vip
new ProductData(){productID = monthlyCardKey,productType = ProductType.subscription},//月卡
});
//注册内购相关事件
ZMYSDKManager.I.Iap_ShowLoading += ShowLoading;
ZMYSDKManager.I.Iap_CloseLoading += CloseLoading;
ZMYSDKManager.I.Iap_ReceiveConsumableCommodity += ReceiveConsumableCommodity;
ZMYSDKManager.I.Iap_ReshNonConsumableState += ReshNonConsumable;
ZMYSDKManager.I.Iap_ReshSubscriptionState += ReshSubscription;
ZMYSDKManager.I.GetQryGivenGiftCallback += GetQryGivenGiftCallback;
//为了测试需要,延迟一会激励视频窗口的出现
StartCoroutine(wait());
m_loading.SetActive(false);
}
IEnumerator wait()
{
yield return new WaitForSeconds(1);
m_storePlan.SetActive(true);
}
public void Click_RestoreBuy()
{
ZMYSDKManager.I.RequestRestoreBuy();
}
public void Click_CheckSubscription()
{
ZMYSDKManager.I.RequestCheckSubscription(monthlyCardKey);
}
void ShowLoading()
{
m_loading.SetActive(true);
}
void CloseLoading()
{
m_loading.SetActive(false);
}
/// 消耗商品
/// <param name="channel"></param>
/// <param name="key"></param>
public void ReceiveConsumableCommodity(CommdityChannel channel, string key)
{
m_resultTxt.text = $"IAP:产品[{key}]购买成功,购买渠道[{channel}]";
m_glob++;
PlayerPrefs.SetInt(globKey, m_glob);
ReshUI();
}
/// 非消耗商品
/// <param name="channel"></param>
/// <param name="key"></param>
public void ReshNonConsumable(CommdityChannel channel, string key)
{
m_resultTxt.text = $"IAP:产品[{key}]购买成功,购买渠道[{channel}]";
if (key.Equals(vipKey))
{
m_vip = true;
PlayerPrefs.GetInt(vipKey, 1);
}
ReshUI();
}
/// 订阅商品
/// <param name="channel"></param>
/// <param name="key"></param>
public void ReshSubscription(NonConsumableState state)
{
m_resultTxt.text = $"IAP:刷新订阅产品[{state.productId}]状态,订阅状态[{state.state}] 时间:[{state.expiresDate}]";
m_mcState = state.state;
m_mcExpiresDate = state.expiresDate;
PlayerPrefs.SetInt(monthlyCardKey, 2);
PlayerPrefs.SetString(monthlyCardKey + "Time", m_mcExpiresDate);
ReshUI();
}
public void Click_QryGivenGift()
{
ZMYSDKManager.I.Sdk.GetQryGivenGiftStatic();
}
void GetQryGivenGiftCallback(List<QryGivenGift> datas)
{
for (int i = 0; i < datas.Count; i++)
{
QryGivenGift item = datas[i];
//模拟发货
m_glob++;
PlayerPrefs.SetInt(globKey, m_glob);
ReshUI();
string tips = $"收到服务器赠送道具:[{item.prodId} 数量:[{item.quantity}]]";
Debug.Log(tips);
m_resultTxt.text = tips;
//通知服务器发货成功
ZMYSDKManager.I.Sdk.UpSendGivenGiftResultStatic(item.orderNo);
}
}
void OnDestroy()
{
ZMYSDKManager.I.Iap_ShowLoading -= ShowLoading;
ZMYSDKManager.I.Iap_CloseLoading -= CloseLoading;
ZMYSDKManager.I.Iap_ReceiveConsumableCommodity -= ReceiveConsumableCommodity;
ZMYSDKManager.I.Iap_ReshNonConsumableState -= ReshNonConsumable;
ZMYSDKManager.I.Iap_ReshSubscriptionState -= ReshSubscription;
ZMYSDKManager.I.GetQryGivenGiftCallback -= GetQryGivenGiftCallback;
ZMYSDKManager.I.CloseIap();
}
}
点我快速对接
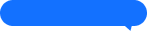

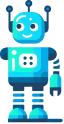